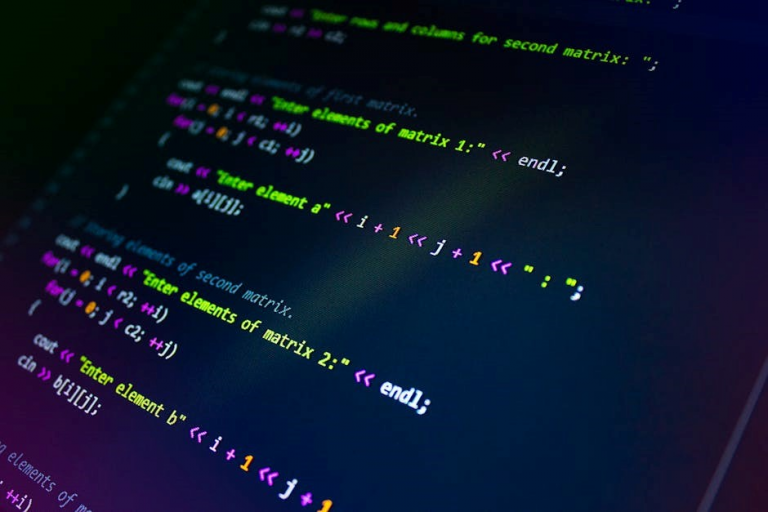
Data structures and algorithms are fundamental to efficient programming in Python‚ enabling scalable and optimized solutions. This section explores their core concepts‚ practical applications‚ and significance in modern computing.
Why Python is Ideal for Learning Data Structures and Algorithms
Python’s simplicity‚ readability‚ and versatility make it an excellent choice for learning data structures and algorithms. Its clean syntax allows beginners to focus on concepts without getting bogged down by complex code. Python’s built-in data structures‚ such as lists‚ dictionaries‚ and sets‚ provide a strong foundation for implementing algorithms. Additionally‚ Python’s extensive libraries and frameworks support advanced operations‚ making it a versatile tool for both education and real-world applications. Its cross-platform compatibility and large community ensure ample resources and support‚ fostering a productive learning environment for students and professionals alike.
Overview of Key Concepts in Data Structures and Algorithms
Data structures and algorithms form the backbone of efficient programming‚ enabling organized data storage and retrieval. Key concepts include arrays‚ linked lists‚ stacks‚ queues‚ trees‚ and graphs‚ each offering unique ways to manage data. Algorithms such as sorting‚ searching‚ and graph traversal provide methods to manipulate and analyze data. Understanding algorithm complexity‚ measured using Big-O notation‚ is crucial for optimizing performance. These concepts are essential for solving real-world problems in fields like data science‚ machine learning‚ and network science. Mastery of these fundamentals allows developers to create efficient‚ scalable‚ and robust solutions‚ balancing time and space complexity effectively.
Fundamental Data Structures in Python
Python implements basic data structures like arrays‚ linked lists‚ stacks‚ queues‚ trees‚ and graphs. These structures organize data efficiently‚ enabling effective storage‚ access‚ and manipulation for various applications.
Arrays and Lists
In Python‚ arrays and lists are foundational data structures for storing collections of elements. Lists are more versatile‚ allowing dynamic resizing and heterogeneous data types. Python’s built-in list data type enables efficient operations like indexing‚ slicing‚ and appending. While Python doesn’t have a built-in array type‚ the array module provides homogeneous storage for primitive data types. Lists are often used interchangeably with arrays due to their flexibility. Common operations include element access (O(1) time)‚ insertion‚ and deletion (O(n) time). Understanding these structures is crucial for implementing more complex data structures and algorithms efficiently in Python.
Linked Lists
A linked list is a linear data structure consisting of nodes‚ each containing data and a reference to the next node. Unlike arrays‚ linked lists store elements non-contiguously. In Python‚ linked lists are not built-in‚ but can be implemented using classes. Singly linked lists have nodes pointing to the next node‚ while doubly linked lists also point to the previous node. Linked lists allow efficient insertions and deletions without shifting elements‚ making them suitable for dynamic applications. However‚ they lack random access‚ requiring O(n) time for element retrieval. Common operations include traversal‚ insertion‚ and deletion‚ with varying time complexities based on the specific implementation and use case.
Stacks and Queues
Stacks and queues are linear data structures that follow specific access patterns. A stack operates on the Last-In-First-Out (LIFO) principle‚ allowing elements to be pushed and popped from the top. Common operations include push‚ pop‚ and peek. Queues‚ on the other hand‚ follow the First-In-First-Out (FIFO) principle‚ with elements enqueued at the rear and dequeued from the front. Both structures are used for efficient data management in algorithms like recursion‚ undo/redo systems‚ and job scheduling. In Python‚ stacks can be implemented using lists‚ while queues can be created with the `queue` module for thread-safe operations‚ ensuring reliable data processing in various applications.
Trees and Graphs
Trees and graphs are non-linear data structures used to represent hierarchical and complex relationships. Trees consist of nodes with parent-child relationships‚ while graphs contain nodes (vertices) connected by edges‚ allowing multiple connections. Common tree types include binary trees‚ B-trees‚ and AVL trees‚ used in databases and file systems. Graphs are categorized as directed or undirected and are essential for network analysis‚ pathfinding‚ and social network modeling. Algorithms like BFS and DFS are used for traversal‚ enabling efficient data retrieval and manipulation. In Python‚ trees and graphs can be implemented using dictionaries‚ classes‚ or libraries like NetworkX‚ making them versatile tools for solving real-world problems in data science and beyond.
Algorithms for Problem Solving
Algorithms are essential for solving complex problems efficiently. They provide step-by-step solutions‚ enabling data organization‚ processing‚ and retrieval. Key algorithms include sorting‚ searching‚ and graph traversal‚ which are fundamental for tackling real-world challenges in computing and data science‚ leveraging Python’s simplicity for effective implementation.
Sorting Algorithms
Sorting algorithms are methods for arranging data elements in a specific order. Common types include Bubble‚ Selection‚ Insertion‚ Merge‚ and Quick Sort. Each has varying time complexities‚ with Merge and Quick Sort being more efficient for large datasets. Understanding these algorithms is crucial for efficient data processing in Python‚ as they form the basis for solving complex problems. They are widely discussed in resources like “Data Structures and Algorithms in Python” by Michael T. Goodrich‚ emphasizing their practical applications in data science and machine learning.
Searching Algorithms
Searching algorithms are techniques used to locate specific data within a collection. Linear Search is a basic method that checks each element sequentially‚ while Binary Search is more efficient for sorted data. These algorithms are essential for efficient data retrieval and are widely used in various applications. Resources like “Data Structures and Algorithms in Python” by Michael T. Goodrich provide in-depth explanations‚ highlighting their importance in programming. Understanding these concepts is crucial for optimizing performance in data-intensive tasks‚ making them a cornerstone of Python programming and problem-solving strategies in fields like data science and machine learning.
Graph Traversal Algorithms
Graph traversal algorithms are methods used to explore each node and edge in a graph exactly once. Common techniques include Breadth-First Search (BFS) and Depth-First Search (DFS). BFS explores nodes level by level‚ using a queue‚ while DFS explores as far as possible along each branch‚ using a stack. These algorithms are essential for tasks like finding the shortest path‚ detecting cycles‚ and identifying connected components. Resources such as “Data Structures and Algorithms in Python” by Michael T. Goodrich provide detailed insights‚ making them invaluable for understanding and implementing these algorithms effectively in Python.
Dynamic Programming
Dynamic programming is a problem-solving approach that breaks complex problems into smaller subproblems‚ solving each only once and storing solutions to subproblems to avoid redundant computation. This method optimizes time and space efficiency‚ making it ideal for problems with overlapping subproblems and optimal substructure. Techniques like memoization and tabulation are used to store intermediate results. Common applications include the knapsack problem‚ shortest path algorithms‚ and matrix chain multiplication. Resources such as “Data Structures and Algorithms in Python” by Michael T. Goodrich provide comprehensive guidance on implementing dynamic programming effectively in Python.
Greedy Algorithms
Greedy algorithms solve problems by making the locally optimal choice at each step‚ hoping it leads to a globally optimal solution. They are efficient and straightforward‚ often used in scenarios like coin change‚ activity selection‚ and graph algorithms. Unlike dynamic programming‚ greedy algorithms do not require storing intermediate results‚ making them memory-efficient. However‚ they may not always yield the correct solution for every problem. Python’s simplicity and built-in data structures make it an ideal language for implementing greedy algorithms. Resources like “Data Structures and Algorithms in Python” provide detailed examples and guidance on mastering these techniques effectively.
Advanced Topics in Data Structures and Algorithms
Explore advanced techniques like hashing‚ recursion‚ and memoization to optimize complex problems. These methods enhance efficiency and scalability‚ crucial for real-world applications and large-scale data handling.
Hashing Techniques
Hashing techniques are essential for efficient data storage and retrieval. Python’s built-in dictionaries utilize hash tables‚ enabling average O(1) time complexity for operations like insertions and lookups. Hash functions map keys to indices‚ ensuring data is distributed uniformly. Collision resolution methods‚ such as chaining or open addressing‚ handle key conflicts. These techniques are vital for applications requiring fast data access‚ like caches and databases; Understanding hashing is crucial for optimizing performance in data-intensive tasks‚ making it a cornerstone in advanced data structure implementations and algorithm design.
Recursion and Memoization
Recursion is a powerful technique where functions call themselves to solve problems by breaking them into smaller subproblems. It is particularly useful for tree traversals‚ factorial calculations‚ and dynamic programming. Memoization optimizes recursive solutions by caching results of expensive function calls‚ reducing redundant computations. In Python‚ memoization can be implemented using decorators like `lru_cache`‚ enhancing performance in algorithms with overlapping subproblems. Together‚ recursion and memoization enable efficient solutions for complex problems‚ leveraging Python’s simplicity and flexibility. These concepts are foundational for advanced algorithm design and problem-solving strategies‚ ensuring scalability and optimal resource utilization in various applications. Their combined use is transformative in handling intricate computational tasks.
Big-O Notation and Algorithm Complexity
Big-O notation is a critical concept for analyzing the performance and scalability of algorithms. It measures the worst-case time or space complexity‚ providing insights into how efficiently an algorithm handles increasing input sizes. Common complexities include O(1) for constant time‚ O(n) for linear time‚ O(n²) for quadratic time‚ and O(log n) for logarithmic time. Understanding algorithm complexity is essential for designing efficient solutions‚ as it helps predict performance and compare different approaches. This section delves into the fundamentals of Big-O notation‚ its application in evaluating algorithms‚ and its role in optimizing data structures and ensuring scalability in Python. Mastery of this concept is vital for any developer aiming to write efficient and scalable code. By analyzing complexity‚ developers can make informed decisions about algorithm selection and optimization‚ directly impacting the overall performance of their applications. Grasping Big-O notation enables the creation of more robust and efficient systems‚ aligning with best practices in software development. This knowledge is particularly valuable in scenarios involving large datasets and high-performance requirements‚ where even slight improvements in complexity can lead to significant gains in execution speed and resource utilization. Ultimately‚ understanding algorithm complexity empowers developers to craft solutions that are not only effective but also scalable and sustainable in the long term. Through practical examples and real-world applications‚ this section provides a comprehensive understanding of Big-O notation and its practical implications in Python programming. By the end of this section‚ readers will be equipped with the tools to critically evaluate and optimize their own algorithms‚ ensuring they meet the demands of modern computing challenges. The ability to analyze and communicate algorithm complexity is a cornerstone skill in computer science‚ and this section serves as a foundational guide to achieving proficiency in this area. Whether working on small-scale scripts or large-scale systems‚ the principles of Big-O notation and algorithm complexity are indispensable for every Python developer striving to write better code. This section underscores the importance of these concepts and provides a clear pathway to mastering them. With a strong grasp of algorithm complexity‚ developers can tackle complex problems with confidence‚ knowing their solutions are both efficient and scalable. The insights gained here will prove invaluable in a wide range of applications‚ from data science and machine learning to web development and system design. By prioritizing algorithm complexity analysis‚ developers can ensure their code is not only functional but also performant‚ meeting the high standards expected in today’s fast-paced technological landscape. This section is a must-read for anyone serious about advancing their skills in Python and algorithm design. The concepts explored here form the bedrock of efficient programming‚ enabling readers to approach problems with a deeper understanding of what makes an algorithm truly effective. Through a combination of theoretical explanation and practical examples‚ this section bridges the gap between abstract ideas and real-world application‚ making it an essential resource for developers at all levels. By mastering Big-O notation and algorithm complexity‚ readers will be well-prepared to address the challenges of modern software development and create solutions that stand the test of time. This section is a testament to the enduring importance of these concepts in the ever-evolving field of computer science‚ offering readers a clear and accessible guide to achieving excellence in algorithm design. The ability to analyze and optimize algorithm complexity is not just a skill—it’s a necessity for any developer aiming to build high-performance‚ scalable‚ and maintainable applications. With this section as their guide‚ readers will gain the knowledge and confidence to take their programming skills to the next level‚ ensuring their work is both innovative and efficient. The principles of Big-O notation and algorithm complexity are timeless‚ and understanding them is key to unlocking the full potential of Python and its applications. This section is a valuable resource for anyone looking to deepen their understanding of these fundamental concepts and apply them effectively in their own projects. By focusing on both theory and practice‚ it provides a holistic approach to mastering algorithm complexity‚ equipping readers with the tools they need to succeed in an increasingly demanding technological environment; The insights and techniques presented here will serve as a reliable foundation for any developer seeking to write efficient‚ scalable‚ and high-performance code in Python. With a clear understanding of Big-O notation and algorithm complexity‚ readers will be empowered to make informed design decisions and create solutions that excel in both functionality and performance. This section is a comprehensive and indispensable guide to achieving algorithmic excellence in Python‚ ensuring that readers are well-prepared to meet the challenges of contemporary software development. The emphasis on practical application and real-world examples makes this section particularly valuable‚ as it bridges the gap between theoretical knowledge and hands-on experience. By the end of this section‚ readers will not only understand the concepts of Big-O notation and algorithm complexity but also be able to apply them effectively in their own work. This ability to translate theory into practice is a hallmark of skilled programming and a key focus of this section. Through its clear explanations‚ engaging examples‚ and practical advice‚ this section demystifies the complexities of algorithm analysis‚ making it accessible to developers of all backgrounds and experience levels. Whether you’re just starting out or looking to refine your skills‚ this section offers insights and techniques that will enhance your approach to algorithm design and problem-solving in Python. The principles discussed here are applicable across a wide range of domains‚ ensuring that readers can apply their knowledge in diverse and dynamic programming environments. By mastering the concepts of Big-O notation and algorithm complexity‚ developers can ensure their solutions are not only effective but also efficient‚ scalable‚ and maintainable. This section is a vital resource for anyone committed to writing high-quality‚ performant code in Python. The ability to analyze and optimize algorithm complexity is a cornerstone of successful software development‚ and this section provides a thorough and engaging guide to achieving this goal. With its focus on clarity‚ practicality‚ and real-world application‚ this section is an essential addition to the toolkit of any serious Python developer. By the end of this section‚ readers will have gained a deeper understanding of algorithm complexity and the skills to apply this knowledge in their own projects‚ leading to better‚ more efficient code. The concepts explored here are fundamental to the art of programming‚ and this section serves as a trusted guide for developers seeking to elevate their craft. Through its comprehensive coverage and emphasis on practical learning‚ this section empowers readers to take control of their algorithm design process‚ ensuring their solutions are optimized for performance and scalability. The insights and techniques presented here will prove invaluable in a wide range of programming scenarios‚ from optimizing data structures to streamlining complex computations. By prioritizing algorithm complexity analysis‚ developers can create solutions that are not only functional but also highly efficient‚ meeting the rigorous demands of modern software development. This section is a testament to the importance of understanding and applying algorithm complexity principles in Python‚ offering readers a clear and actionable path to achieving algorithmic excellence. The ability to analyze and optimize algorithm complexity is not just a skill—it’s a necessity for any developer aiming to build high-performance‚ scalable‚ and maintainable applications. With this section as their guide‚ readers will gain the knowledge and confidence to take their programming skills to the next level‚ ensuring their work is both innovative and efficient. The principles of Big-O notation and algorithm complexity are timeless‚ and understanding them is key to unlocking the full potential of Python and its applications. This section is a valuable resource for anyone looking to deepen their understanding of these fundamental concepts and apply them effectively in their own projects. By focusing on both theory and practice‚ it provides a holistic approach to mastering algorithm complexity‚ equipping readers with the tools they need to succeed in an increasingly demanding technological environment. The insights and techniques presented here will serve as a reliable foundation for any developer seeking to write efficient‚ scalable‚ and high-performance code
Applications of Data Structures and Algorithms in Python
Data structures and algorithms in Python are crucial for data science‚ machine learning‚ and big data‚ enabling efficient solutions to real-world problems and scalability.